AP Computer Science A: Unit 1
Unit 1.1 Video 1 Notes (Skill 2.B)-
System/class methods
- Block comments: the compiler will ignore any text between the /* and */ characters. (Name, date, purpose.)
- Class declaration: identifies the name, the start, and the end of the class. The class name MUST match the file name. (i.e., HelloWorld.java 🡪 public class HelloWorld{)
- Main method: controls all of the action in the program (ex: public static void main (String[] args){).
- System.out: object that generate output to the console (ex: System.out.print / System.out.println).
Key Takeaways-
- When we want output to go to the console, call System class methods to generate output to the console.
- System.out.print and System.out.println display information on the computer monitor.
- System.out.println moves the cursor to a new line after the information has been displayed while System.out.print does not.
Unit 1.1 Video 2 Notes (Skill 2.B)-
String literals
- Literally = verbatim, word for word, to the letter, exactly, precisely.
- A string literal in java is an exact sequence of characters (letters, numbers, or symbols) which are enclosed between two quotation marks. Ex: “This is a string literal.” “ABC123#$%abc.”
Unit 1.1 Video 3 Notes (Skill 4.B)-
Syntax errors and logic errors
- Compiler: translates source code into a language that computer can understand and executes (sends output back) to the computer.
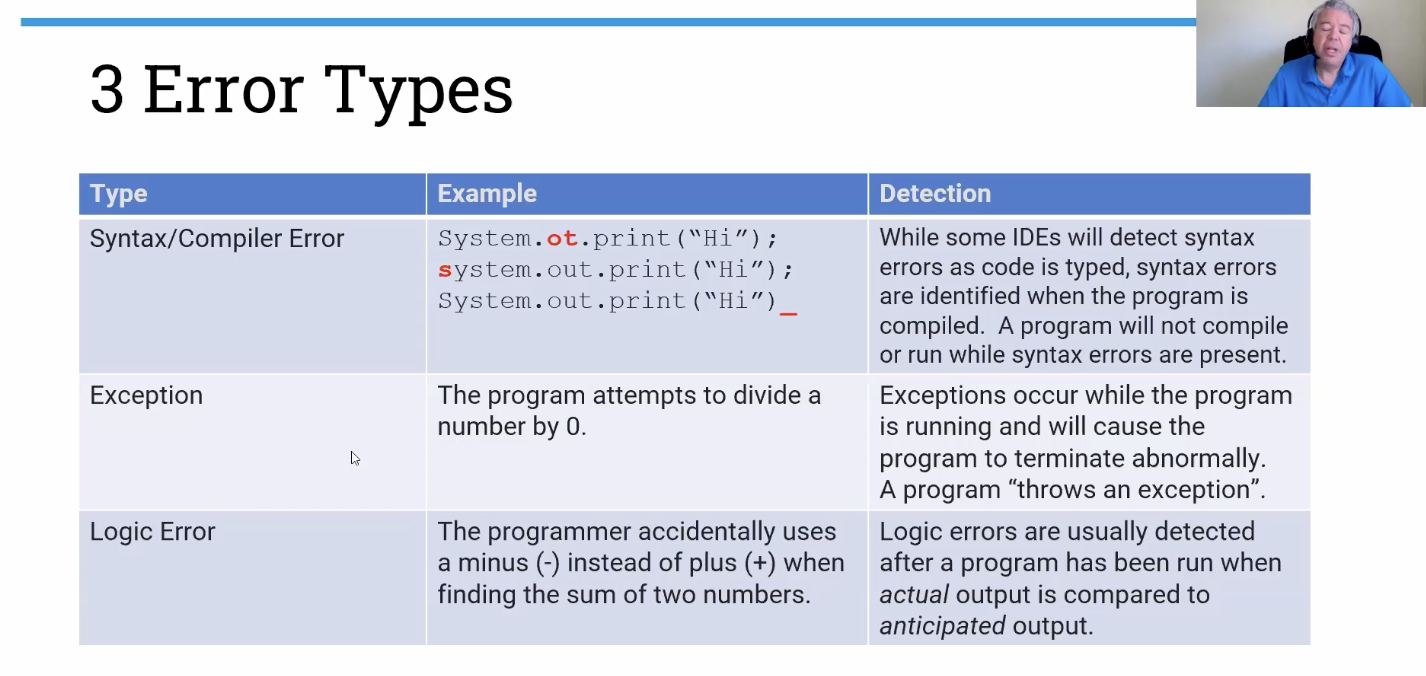
- Syntax error examples: system.out.prnt, (“A Rocks);, etc.
- Logic error example: incorrect usage of System.out.println.
Unit 1.2 Video 1 Notes (Skill 1.A)-
Variables and Data Types
- Primitive Data: Determines the size and type of information that we can work with in a Java program. Ex: Boolean (true of false), int (whole numbers), double/floating-point values (decimal numbers).
- Non-primitive types can use methods to perform actions, but primitive types cannot. Except for String[s], non-primitive types are generally created by the programmer. A String is called Reference Data.
Unit 1.2 Video 2 Notes (Skill 1.B)-
Variables and Data Types
- Variable: Name given to a memory location that is holding a specified type of value.
- To name a variable… 1) May consist of letters, digits, or an underscore (case sensitive), 2) May not start with a digit, 3) Spaces are not allowed, 4) May not use any other characters such as & or @ or $, 5) May not use Java reserved words, 6) Must be in “camelCase.”
- To declare a variable… datatype variableName; or int total; or double intRate or boolean giveBonus;
- What if we want to declare a variable that cannot be changed once we give it a value? A CONSTANT? We use the Java reserved word final in front of the declaration. Ex: final double PI; or final int DAYS_IN_WEEK; or final boolean JAVA_ROCKS. Note: For final variables (constant), we must use ALL CAPS and underscores when we name them. When a variable is declared final, its value cannot be changed once it is initialized.
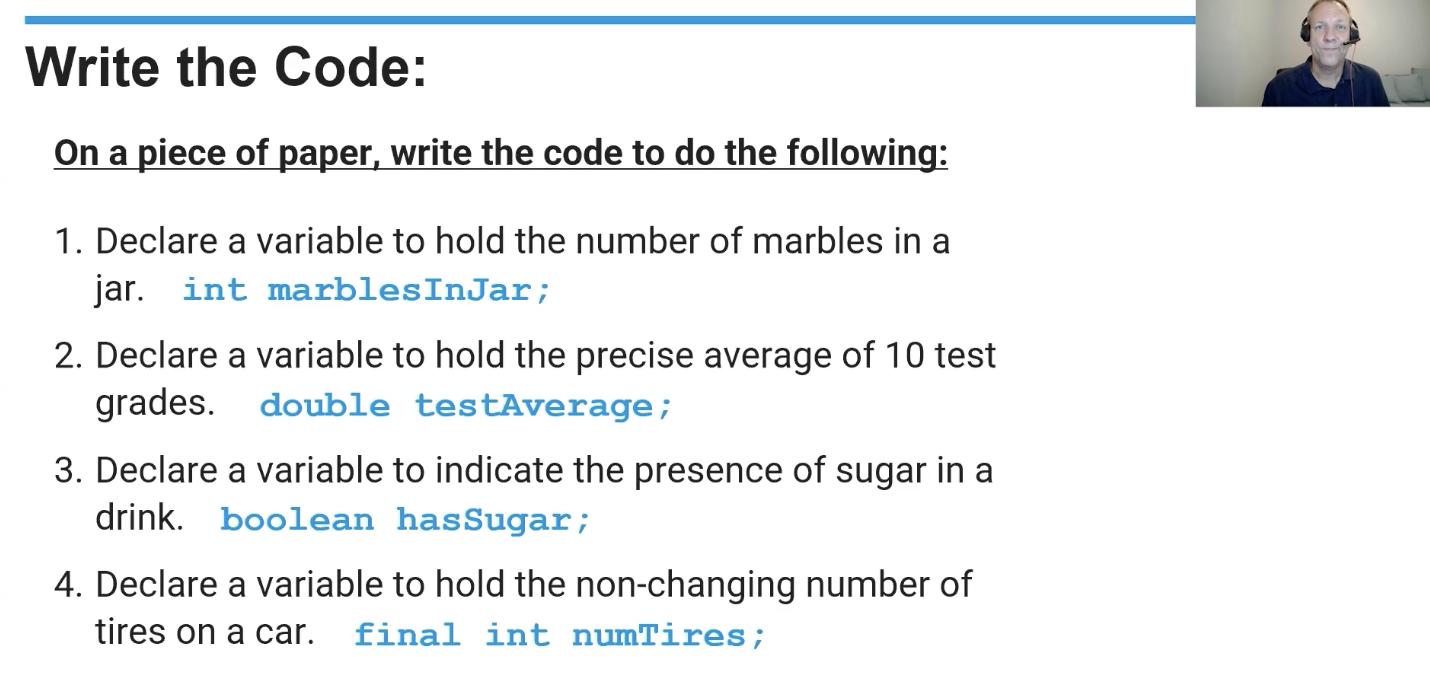
Unit 1.3 Video 1 Notes (Skill 2.A)-
Expressions and Assignment Statements (Basic Arithmetic Knowledge)
- Literal: The source code representation of a fixed value. Ex: 3. System.out.println(3+3);, output is 6.
- String Literal (1.1): Enclosed in double quotes. Ex: “3”. System.out.println(“3” + “3”);, output is 33 (concatenation).
- Basic Arithmetic Operators: +, -, *, /, % (modulus/mod).
- Modulus: Always gives remainder of division problem. Ex: 17/5 (do long division!). 5 goes into 17 three times (15). Subtract (17-15). Remainder is 2. So, 17/5 = 3.4, and 17 % 5 = 2.
Unit 1.3 Video 2 Notes (Skill 2.A)-
Expressions and Assignment Statements (Evaluating Compound Expressions)
- Operator Precedence: PEMDAS and left to right! Ex:
8 * 6 - 14 % 3 + 8 / 2
48 – 14 % 3 + 8 / 2
48 – 2 + 8 / 2
48 – 2 + 4
46 + 4
50
Ex 2:
8 * 6 - 14 % (3 + 8) / 2
8 * 6 - 14 % 11 / 2
48 - 14 % 11 / 2
48 – 3 / 2
48 – 1
47
- An attempt to divide by an integer by zero will result in an ArithmeticException to occur.
Unit 1.3 Video 3 Notes (Skill 1.B)-
Expressions and Assignment Statements (Initialize/Change Stored Values in Variables)
- Equal Sign (=): An instruction. Assigns the value on the right of the assignment operator to the variable on the left. Ex: x = 7; or y = 8 + 4 * x;. CANNOT write 7 = x; because it is a literal value (number that already has its own value).
- Operator Precedence: Equals sign is always the last step in PEMDAS. With the assignment operator, we work from right to left. Takes calculated value from the right and moves it over from the left.
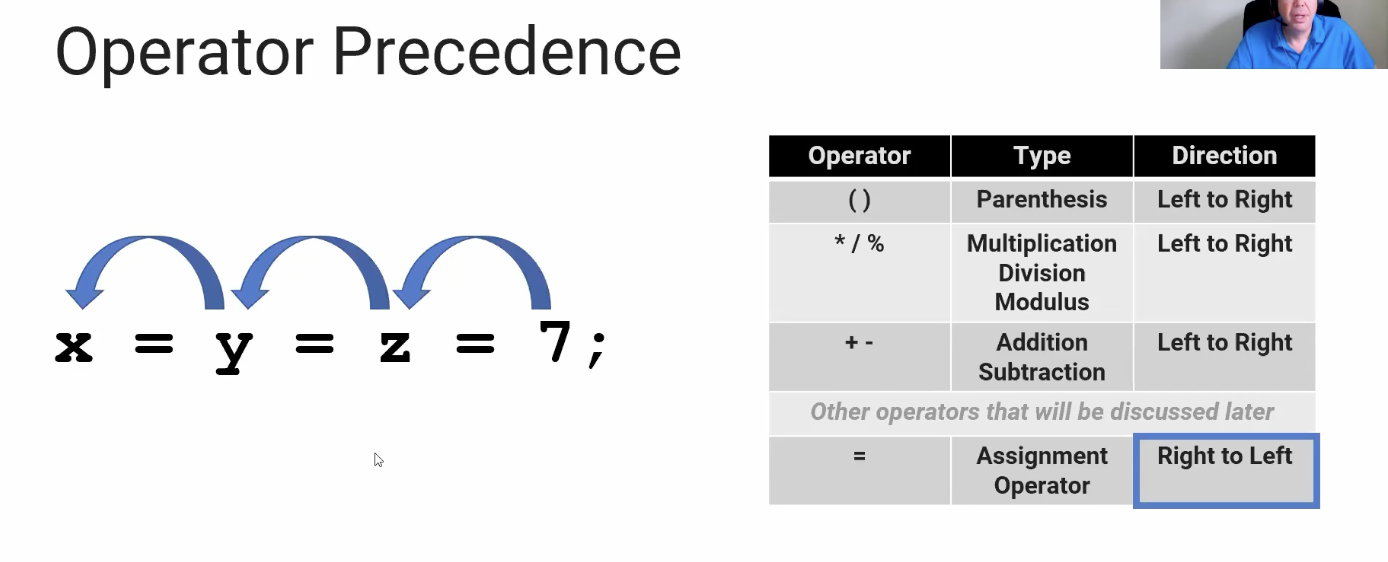
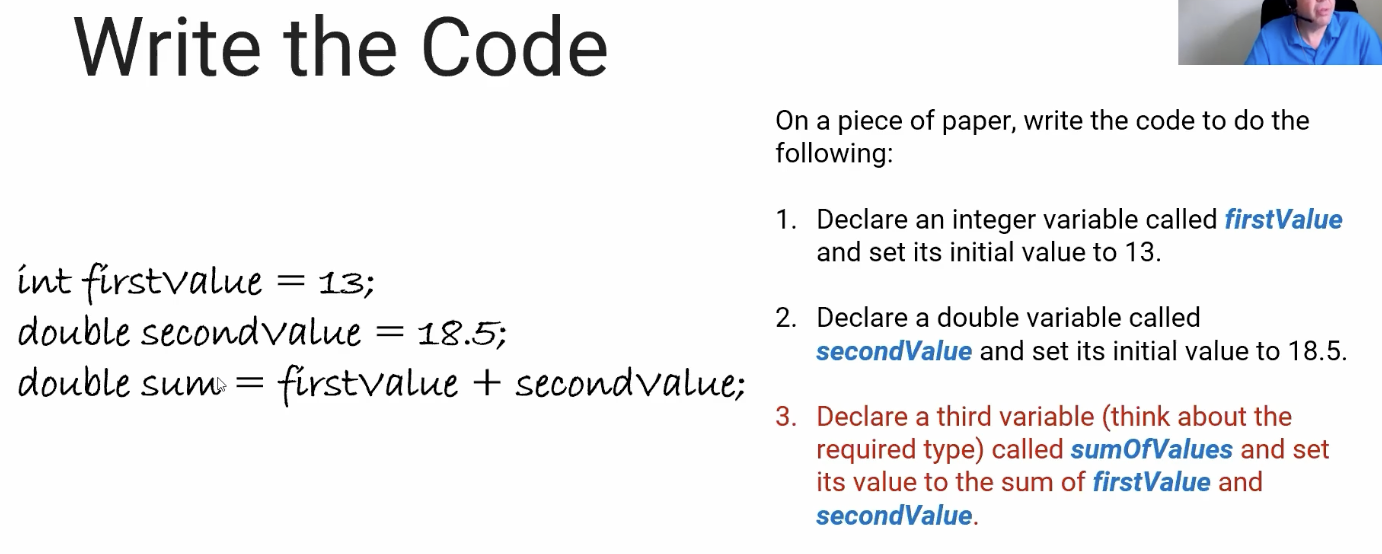
Unit 1.4 Video 1 Notes (Skill 2.B)-
Compound Assignment Operators
Ex: x += 7. Instructions: 1) Take the value currently stored n x. 2) Add 7. 3) Assign the result back to x.
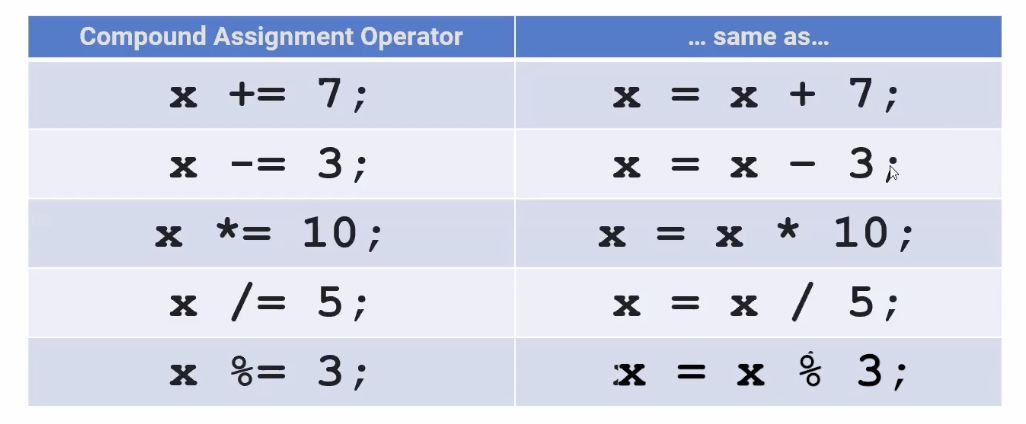
- Tracing the code: becoming the compiler!
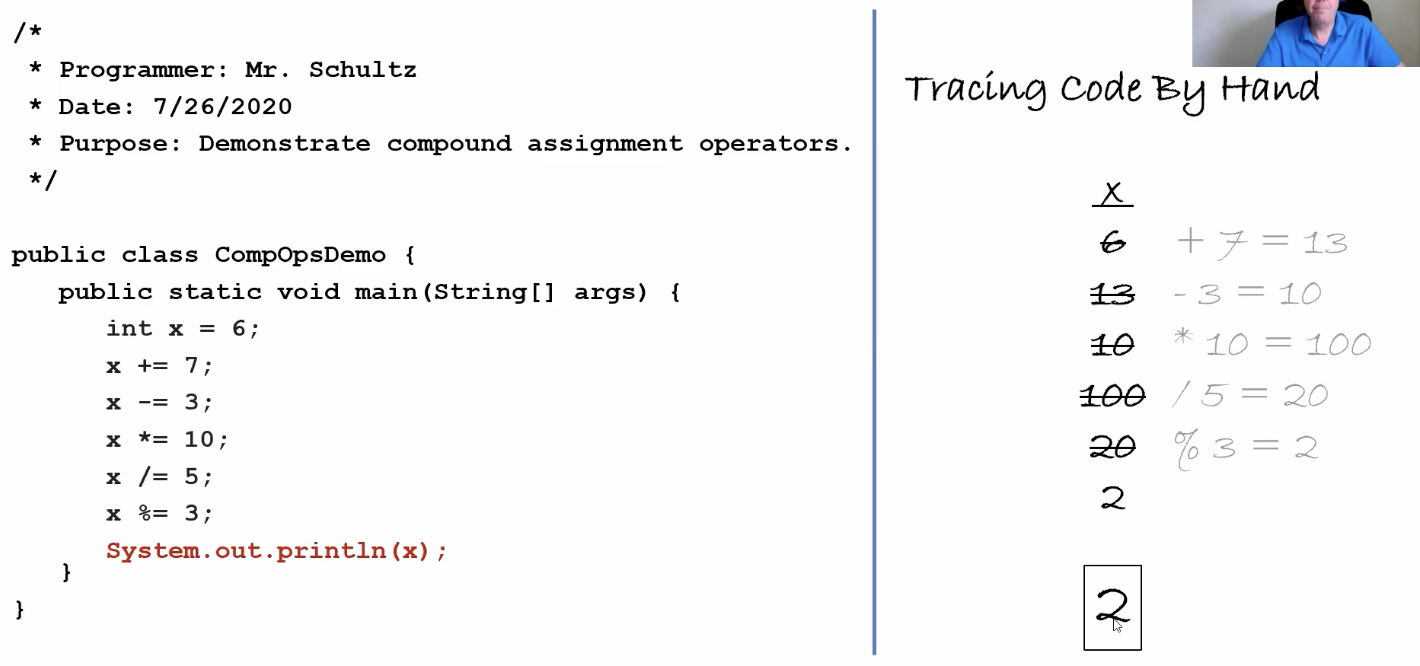
Unit 1.4 Video 2 Notes (Skill 2.B)-
Compound Assignment Operators (increment ++ and decrement --)
Ex: x++; Instruction: 1) Take the value currently stored in x. 2) Add 1. 3) Assign the result back to x. EXCLUSION STATEMENT: The use of increment and decrement operators in the prefix form (i.e., ++x) and inside other expressions (i.e., arr[x++]) is outside the score of this course and the ap exam).

- Tracing the code: becoming the compiler!
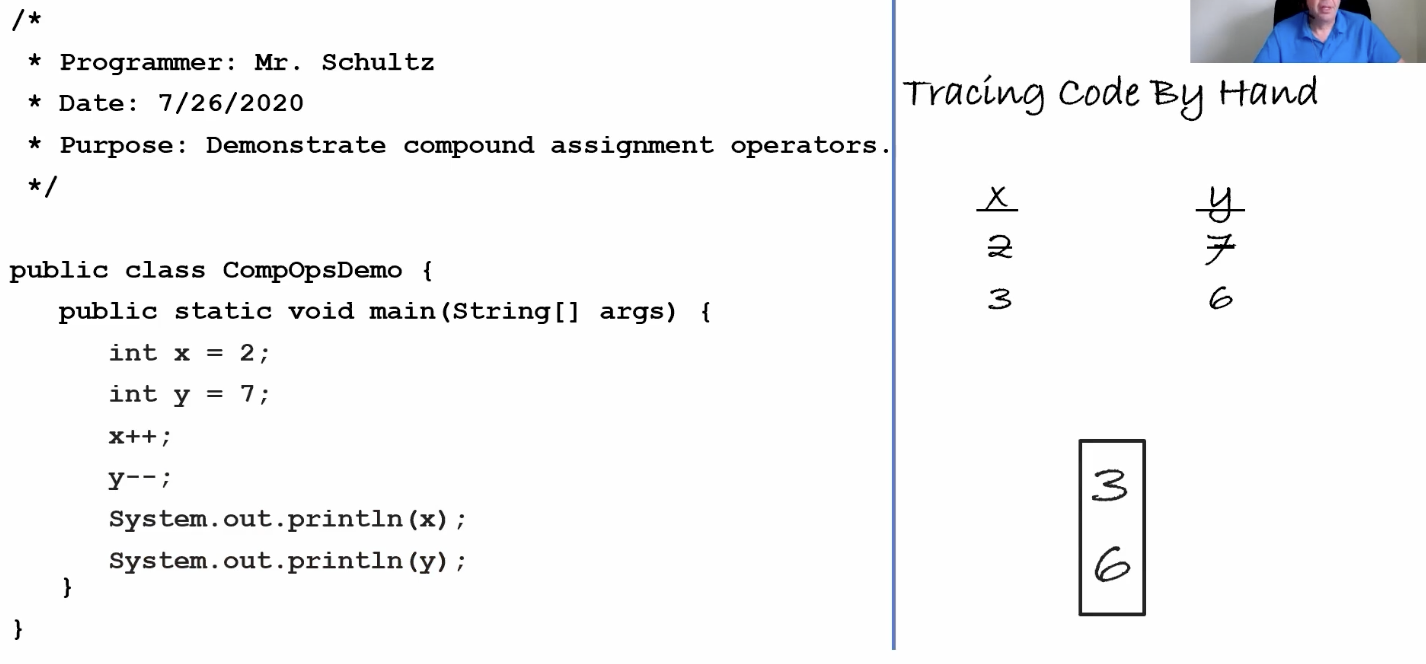
- The increment operator (==) and decrement operator (--) are used to add 1 or subtract 1 from a stored value or variable or an array element. The new value is assigned to the variable or array element.
Unit 1.4 Video 3 Notes (Skill 5.A)-
Compound Assignment Operators (describing the behavior and overall purpose of code)
int x = 23; 1) define an int var x, set its initial value to 23
x *= 2; 2) take the current value of x, mult. by 2, assign result to x
x %= 10; 3) take the curr. value of x, find x mod 10, assign result to x
System.out.println(x); 4) display the current value of x
Unit 1.5 Video 1 Notes (Skill 2.B)-
Casting and Ranges of Variables
- Casting: used in Java to change the data type of a variable from one type to another.
- Ex:
(int) (2.5 * 3.0) 7
(double) 25 / 4 6.25
6 / (double) 5 1.2
(int) (12 / 5) 2
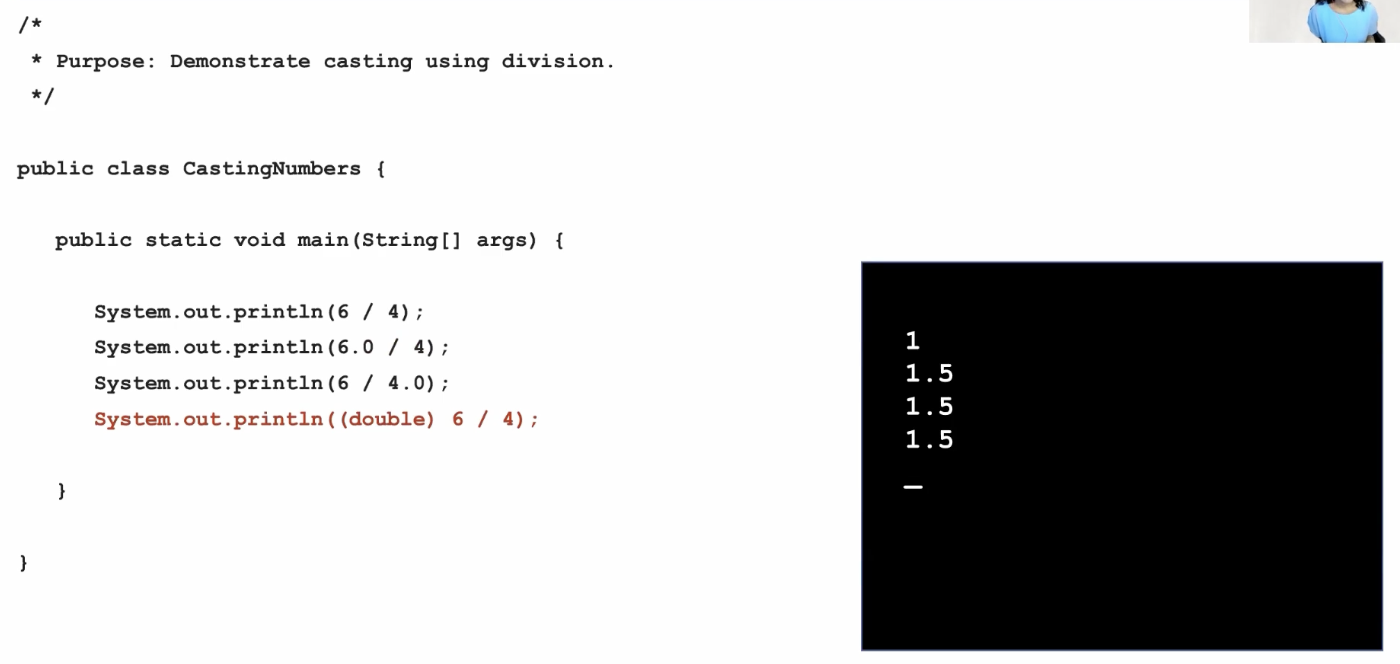
Unit 1.5 Video 2 Notes (Skill 5.B)-
Casting and Ranges of Variables
- Int: 2147483647 (Integer.MAX_VALUE)
-2147483647 (Integer.MIN_VALUE)
Double: up to 14-15 digits.
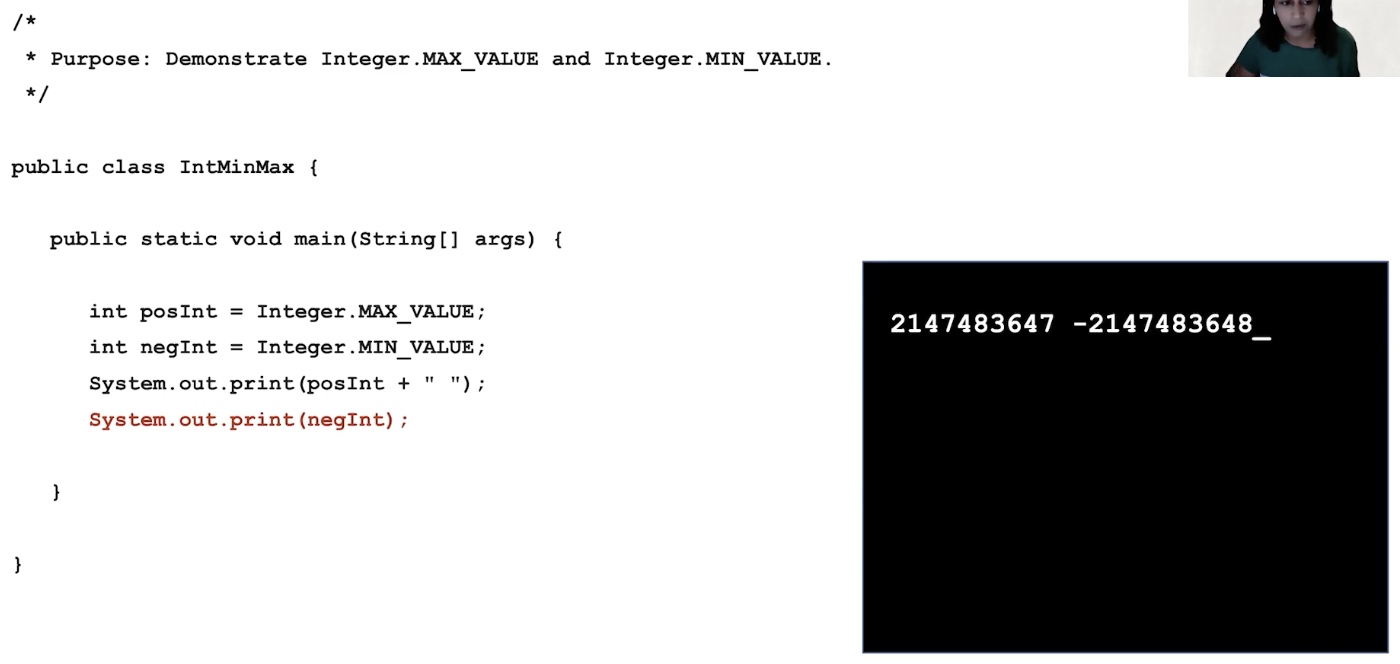
^^ Above image is outside of allowable range (provides unexpected result).